One of the main uses of cross product is in determining the relative position of points and other objects. For this, we define the function orient(A,B,C)=→AB×→AC. It is positive if C is on the left side of →AB, negative on the right side, and zero if C is on the line containing →AB.
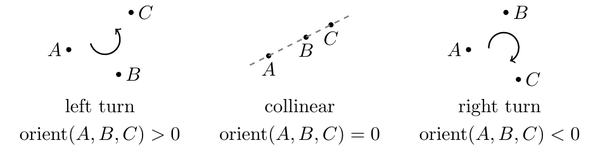
It is straightforward to implement:
// create the vector from point a to point b static Point vec(Point a, Point b) { return new Point(b.x - a.x, b.y - a.y); } static double orient(Point a, Point b, Point c) { return cross(vec(a, b), vec(a, c)); }
In most situations we only care about the sign of orient(a,b,c). Therefore we define a function sorient(a,b,c) which returns the sign of orient(a,b,c)
sorient(a,b,c)={1orient(a,b,c)>00orient(a,b,c)=0−1orient(a,b,c)<0
static double sorient(Point a, Point b, Point c) { double o = orient(a, b, c); return o < 0 ? -1 : o > 0 ? 1 : 0; }